(クラス)
public abstract StringInterface
文字列に対する抽象クラス。
説明
StringInterface は、Curl における文字列クラスに対するインターフェイスです。つまり、
StringInterface は、Curl におけるほどんどの文字列クラスの基礎となるアクセッサとメソッドを備えています。
StringInterface は抽象クラスなので、インスタンス化することはできません。文字列オブジェクトを作成するには、
StringInterface から継承される文字列クラスのいずれかをインスタンス化します。Curl では
String クラスの使用が目的に応じて最適化されます。読み取り専用文字列を使用可能な場合でも、このクラスを使ってください。
注意事項
以下に、Curl の文字列クラスの階層を示します。
アクセサ public StringInterface.empty?:
bool
アクセサ public StringInterface.for-loop-count:
int
アクセサ public abstract StringInterface.size:
int
public | {StringInterface.compare}:int |
public | {StringInterface.equal?}:bool |
find: | self を検索し、特定の文字 c があるかどうかを調べます。 |
public | {StringInterface.find}:int |
public | {StringInterface.find-char-class}:int |
public | {StringInterface.find-string str:StringInterface,ignore-case?:bool = false,search-direction:SearchDirection = SearchDirection.forward,starting-index:int = {if search-direction == SearchDirection.forward then
0
else
{max -1, self.size - str.size}
} }:int |
public abstract | {StringInterface.get index:int}:char |
prefix?: | self の先頭が str である場合、true を返します。 |
public | {StringInterface.prefix?}:bool |
public final | {StringInterface.replace-clone}:String |
split: | self を、指定された文字の出現個所で分割します。 |
public | {StringInterface.substr start:int, length:int}:String |
suffix?: | self の末尾が str である場合、true を返します。 |
public | {StringInterface.suffix?}:bool |
tail: | {self.substr start, self.size - start} を返します。 |
public | {StringInterface.tail start:int}:String |
public | {StringInterface.to-double whitespace:CharClass = CharClass.whitespace-chars,start:int = 0,error-if-over-or-underflow?:bool = true }:(
val:double,
n-chars-consumed:int,
overflow-or-underflow?:bool
) |
public | {StringInterface.to-int radix:int = 0,start:int = 0,whitespace:CharClass = CharClass.whitespace-chars,error-if-overflow?:bool = true }:(
val:int,
n-chars-consumed:int,
overflow?:bool,
radix:int
) |
public | {StringInterface.to-int64 radix:int = 0,start:int = 0,whitespace:CharClass = CharClass.whitespace-chars,error-if-overflow?:bool = true }:(
val:int64,
n-chars-consumed:int,
overflow?:bool,
radix:int
) |
public | {StringInterface.to-lower-clone}:String |
public | {StringInterface.to-String}:String |
public | {StringInterface.to-upper-clone}:String |
trim-clone: | self のクローンを返します。その際、文字列の左端と右端の両方がトリミングされます。 |
public | {StringInterface.trim-clone trim-chars:CharClass = CharClass.whitespace-chars }:String |
public | {StringInterface.trim-left-clone trim-chars:CharClass = CharClass.whitespace-chars }:String |
public | {StringInterface.trim-right-clone trim-chars:CharClass = CharClass.whitespace-chars }:String |
(アクセサ)
アクセサ public StringInterface.empty?:
bool self が空であるかどうかを検査します。
戻り値
bool 値。self の中に文字がまったくない場合、true を返します。それ以外の場合は、false を返します。
例
次の例は、文字がまったく含まれない文字列の場合です。
例 |
 |
{value
|| Declare and initialize an empty string
let s:String = ""
|| Check if the string is empty and display an
|| appropriate message
{if s.empty? then
{text The string is empty!}
else
{text The string has characters!}
}
}
| |
次の例は、文字を含む文字列の場合です。
例 |
 |
{value
|| Declare and initialize a string
let s:String = "Hello World!"
|| Check if the string is empty and display an
|| appropriate message
{if s.empty? then
{text The string is empty!}
else
{text The string has characters!}
}
}
| |
(アクセサ)
アクセサ public StringInterface.for-loop-count:
int 文字列に対して繰り返し処理を実行するコンテナである for ループが出現したときに Curl コンパイラが使うゲッター。
注意事項
このゲッターを直接呼び出さないでください。このゲッターは、Curl コンパイラによってのみ使われるよう用意されているものです。
(アクセサ)
アクセサ public abstract StringInterface.size:
int self 内の文字数。
戻り値
文字数を示す int 値。
説明
読み取り専用文字列の場合
書き込み可能文字列の場合
- 文字列内の文字数を返します。
- 文字列内の文字数を設定できます。
size をその文字列の現在のサイズよりも小さい値に設定した場合、その文字列は切り詰められます。
size を現在のサイズよりも大きい値に設定した場合、差分の文字数がその文字列の末尾に追加されます。この場合、追加される文字の値は Unicode 値の
0000 になります。
例
次の例は、読み取り専用文字列に対して
size を使用した場合です。
例 |
 |
{value
|| Declare and initialize a read-only string
let s:String = "Hello World!"
|| Output a message that includes the return value of
|| a call to "size".
|| Note that you must use "value" to display the value
|| of "s.size", rather than the text "s.size".
{text There are {value s.size} characters in the string.}
}
| |
次の例は、書き込み可能文字列に対して
size を使用した場合です。
例 |
 |
{value
|| Declare and initialize a writable string.
let sb:StringBuf = {StringBuf "Hello World!"}
|| Set the size of the string to 5 characters.
|| The string should now contain only the first five
|| characters, "Hello".
set sb.size = 5
|| Output a message that includes the new string
{text If {monospace size} is set to 5, the string
becomes: {value sb}}
}
| |
例 |
 |
{value
|| Declare and initialize a writable string.
let sb:StringBuf = {StringBuf "Hello World!"}
|| Set the size of the string to 20 characters.
|| The string should now have 8 characters
|| with the Unicode value 0000 at the end.
set sb.size = 20
|| Output a message that includes the new string.
{text Then, if {monospace size} is set to 20, the string
becomes: {value sb}}
}
| |
注意事項
(メソッド)
この項目はサポートされていません。内部使用限定となっています。
(メソッド)
public | {StringInterface.compare}:int |
self を、指定された StringInterface と比較します。
ignore-case?: 比較時に大文字と小文字の区別を無視するかどうかを示すキーワード引数。既定では、大文字と小文字の区別は無視されません (ignore-case? = false)。つまり、既定では大文字と小文字は区別されます。たとえば、"aaaa" は "BBBB" よりも大きいと評価されます。
ignore-case? はキーワード引数です。ignore-case? を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:ignore-case? = true)。
ignore-case? に true を設定した場合、比較時に大文字と小文字は区別されません。この場合、"aaaa" は "BBBB" よりも小さいと評価されます。
戻り値
int 型の値。
- self が str よりも小さい場合、-1 を返します。
- self が str と等しい場合、0 を返します。
- self が str よりも大きい場合、1 を返します。
説明
例
例 |
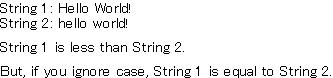 |
{value
|| Declare and initialize two strings
let s1:String = "Hello World!"
let s2:String = "hello world!"
|| Display the strings.
{text String 1: {value s1}
{br}String 2: {value s2}
|| Compare the two strings and display an
|| appropriate message.
{switch {s1.compare s2}
case -1 do
{text String 1 is less than String 2.}
case 0 do
{text String 1 is equal to String 2.}
else
{text String 1 is greater than String 2.}
}
|| Compare the two strings, this time ignoring case,
|| and display an appropriate message.
But, if you ignore case,
{switch {s1.compare s2, ignore-case? = true}
case -1 do
{text String 1 is less than String 2.}
case 0 do
{text String 1 is equal to String 2.}
else
{text String 1 is greater than String 2.}
}
}
}
| |
(メソッド)
public | {StringInterface.equal?}:bool |
self と、指定された StringInterface が等しいかどうかを検査します。
ignore-case?: 検査時に大文字と小文字の区別を無視するかどうかを示すキーワード引数。既定では、大文字と小文字の区別は無視されません (ignore-case? = false)。つまり、既定では大文字と小文字は区別されます。たとえば、"abcdefg" と "ABCDEFG" は等しくありません。
ignore-case? はキーワード引数です。ignore-case? を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:ignore-case? = true)。
ignore-case? に true を設定した場合、検査時に大文字と小文字は区別されません。この場合、"abcdefg" と "ABCDEFG" は等しいと評価されます。
戻り値
bool 値。self 内の各文字が str 内の対応する文字と等しい場合、true を返します。それ以外の場合は、false を返します。
例
例 |
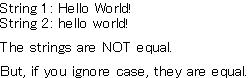 |
{value
|| Declare and initialize two strings
let s1:String = "Hello World!"
let s2:String = "hello world!"
|| Display the strings.
{text
String 1: {value s1}
{br}String 2: {value s2}
|| Test if the two strings are equal and display
|| an appropriate message.
{if {s1.equal? s2} then
{text The strings are equal.}
else
{text The strings are NOT equal.}
}
|| Test if the two strings are equal, this time
|| ignoring case, and display an appropriate message.
But, if you ignore case,
{if {s1.equal? s2, ignore-case? = true} then
{text they are equal.}
else
{text they are NOT equal.}
}
}
}
| |
(メソッド)
public | {StringInterface.find}:int |
self を検索し、特定の文字 c があるかどうかを調べます。
c: 検索する文字。
ignore-case?: 比較時に大文字と小文字の区別を無視するかどうかを示すキーワード引数。
starting-index: 検索を開始する文字のインデックスを示す int 値。左から右に検索する場合、このパラメータの既定値は 0 です。右から左に検索する場合、このパラメータの既定値は self.size - 1 です。
戻り値
一致した文字のインデックスを示す int 値。一致する文字がなかった場合は、-1 を返します。
説明
self を検索し、最初に出現する
c に等しい文字を見つけます。その文字のインデックスを返します。一致する文字がなかった場合は、
-1 を返します。
starting-index が範囲外を指している場合は、
ArrayBoundsException をスローします。
(メソッド)
public | {StringInterface.find-char-class}:int |
self を検索し、char-class 内の文字があるかどうかを調べます。
starting-index: 検索を開始する文字のインデックスを示す int 値。左から右に検索する場合、このパラメータの既定値は 0 です。右から左に検索する場合、このパラメータの既定値は self.size - 1 です。
戻り値
最初に一致した文字のインデックスを示す int 値。一致する文字がなかった場合は、-1 を返します。
説明
self を検索し、
char-class 内にある文字のうち最初に出現する文字を見つけます。その文字のインデックスを返します。一致する文字がなかった場合は、
-1 を返します。
starting-index が範囲外を指している場合は、
ArrayBoundsException をスローします。
(メソッド)
public | {StringInterface.find-string str:StringInterface,ignore-case?:bool = false,search-direction:SearchDirection = SearchDirection.forward,starting-index:int = {if search-direction == SearchDirection.forward then
0
else
{max -1, self.size - str.size}
} }:int |
self を検索し、部分文字列 str があるかどうかを調べます。
str: 検索する部分文字列。
ignore-case?: 比較時に大文字と小文字の区別を無視するかどうかを示すキーワード引数。
starting-index: 検索を開始する文字のインデックスを示す int 値。左から右に検索する場合、このパラメータの既定値は 0 です。右から左に検索する場合、このパラメータの既定値は {max -1, self.size - str.size} です。
戻り値
最初に一致した文字のインデックスを示す int 値。一致する文字列がなかった場合は、-1 を返します。
説明
self を検索し、
str に等しい部分文字列のうち最初に現れるものを見つけます。一致した部分文字列の先頭文字のインデックスを返します。一致する部分文字列がなかった場合は、
-1 を返します。
starting-index または
starting-index +
str.size が範囲外を指している場合は、
ArrayBoundsException をスローします。
(メソッド)
public abstract | {StringInterface.get index:int}:char |
self 内の指定された文字を返します。
index: 取得する文字の位置。最左端の文字の位置は 0 です。最右端の文字の位置は (self.size - 1) です。有効値は 0 ~ (self.size - 1) の範囲です (0 と (self.size - 1) を含む)。
例
例 |
 |
{value
|| Declare and initialize a string.
let s:String = "Hello World!"
|| Display the string and return the
|| character at position 7.
|| Remember that the leftmost position
|| is 0 (zero).
{text {value s}
{br}The character at position 7 is {s.get 7}.}
}
| |
注意事項
(メソッド)
public | {StringInterface.prefix?}:bool |
self の先頭が str である場合、true を返します。
(メソッド)
public final | {StringInterface.replace-clone}:String |
old の各オカレンスが new に置換される場合に新しい String を返します。
old: new で置換する部分文字列。
new: old の各オカレンスを置換する部分文字列。
説明
左から右に部分文字列 old を検索します。各オカレンスごとに、old を削除し、new をつなぎ合わせて、新規に挿入された文字の後から検索を再開します。
例
例 |
 |
{value
|| Declare and initialize a String.
let x:String = "Silly willy"
|| Replace each occurrence of "ill" with "ick".
let y:String = {x.replace-clone "ill", "ick"}
|| Display the resulting String.
y
}
| |
(メソッド)
self を、指定された文字の出現個所で分割します。
split-chars: この文字の出現個所で
self を分割します。
split-chars の既定値は、現在のロケールに対する空白 (
CharClass.whitespace-chars で定義) を意味する文字群です。このメソッドの呼び出しに対して既定値以外の文字を指定するには、その文字を含む
CharClass を指定します。
split-chars はキーワード引数です。
split-chars を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:
split-chars = {CharClass " .,;:"})。
split-chars のキーワード引数に対して
null を指定した場合、このメソッドは、各文字に対する要素が 1 つずつ存在する配列を返します。
戻り値
説明
self を
split-chars 内の各文字の出現個所で分割します。つまり、より短い文字列が複数個作成されます。分割後の文字列の中には
split-chars 内の文字は含まれません。このメソッドは、分割後の文字列からなる配列を返します。
次の場合、このメソッドは空の文字列から成る要素を配列に追加します。
- split-chars 内のいずれかの文字が self の先頭に出現した場合。
- split-chars 内の文字が 2 つ以上連続して出現した場合。
- split-chars 内のいずれかの文字が self の末尾に出現した場合。
split-chars が
null の場合、このメソッドは各文字に対する要素が 1 つずつ存在する配列を返します。
例
例 |
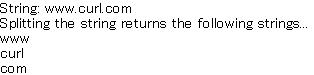 |
{value
|| Declare and initialize a string.
let s:String = "www.curl.com"
|| Split the string at the periods.
let a:StringArray = {s.split split-chars = "."}
|| Declare and instantiate a VBox for displaying
|| an output message indicating the results.
let out:VBox = {VBox}
|| For each element in the results array, add it
|| to the output message.
{for str in a do
{out.add str}
}
|| Display the string and the output message.
{text String: {value s}
{br}Splitting the string returns the following strings...
{br}{value out}
}
}
| |
(メソッド)
public | {StringInterface.substr start:int, length:int}:String |
self の指定された部分文字列を返します。
start: self 内における部分文字列の開始位置。最左端の文字の位置は 0 です。最右端の文字の位置は (self.size - 1) です。有効値は 0 ~ (self.size - 1) の範囲です (0 と (self.size - 1) を含む)。
length: 部分文字列の長さ。つまり、部分文字列内の文字数です。
戻り値
self の指定された部分文字列が格納された
String。この部分文字列は、
start の位置から始まる
length 個の連続した文字です。
注意事項
例
例 |
 |
{value
|| Declare and initialize s1 (the original string).
let s1:String = "Hello World!"
|| Initialize s2 with a substring of s1. The
|| substring begins at position 6 and is 5
|| characters long ("World").
|| Remember that the leftmost position
|| is 0 (zero).
let s2:String = {s1.substr 6, 5}
|| Display the contents of s2.
{value s2}
}
| |
注意事項
クローン作成の詳細については、『Curl 開発者ガイド』の「
文字列クローンの操作」のセクションを参照してください。
(メソッド)
public | {StringInterface.suffix?}:bool |
self の末尾が str である場合、true を返します。
(メソッド)
public | {StringInterface.tail start:int}:String |
{self.substr start, self.size - start} を返します。
(メソッド)
public | {StringInterface.to-double whitespace:CharClass = CharClass.whitespace-chars,start:int = 0,error-if-over-or-underflow?:bool = true }:(
val:double,
n-chars-consumed:int,
overflow-or-underflow?:bool
) |
self を解析して double 型にします。
whitespace:
self の先頭にある、空白を構成する文字。空白文字は通常、スペースとタブです。
whitespace の既定値は、現在のロケールに対する空白 (
CharClass.whitespace-chars で定義) を意味する文字群です。このメソッドの呼び出しに対して既定値以外の空白文字を指定するには、その空白文字を含む
CharClass を指定します。
whitespace はキーワード引数です。
whitespace を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:
whitespace = {CharClass " .,;:"})。空白文字以外にも無視したい文字がある場合、このパラメータを使ってその文字を指定できます。
start:
self 内の解析開始位置。最左端の文字の位置は
0 です。最右端の文字の位置は
(self.size - 1) です。有効値は
0 ~
self.size の範囲です (
0 と
self.size を含む)。既定値は
0 です。
start はキーワード引数です。
start を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:
start = 4)。
start が範囲外を指している場合、このメソッドは
ArrayBoundsException をスローします。
error-if-over-or-underflow?: 数値がオーバーフローまたはアンダーフローしたときにこのメソッドがエラーを生成するかどうかを示す、ブール値のフラグ。数値が大きすぎて
double に格納できない場合、その数値はオーバーフローします。数値が小さすぎて
double に格納できない場合、その数値はアンダーフローします。
error-if-over-or-underflow? を
true に設定した場合、このメソッドはオーバーフローまたはアンダーフローを検出したときにエラーをスローします。エラーが発生するとプログラムの実行が停止し、エラー メッセージが表示されます。既定では、
error-if-over-or-underflow? は
true です。
error-if-over-or-underflow? を
false に設定した場合、このメソッドはエラーを生成せず、次のとおりに値を返します。
- このメソッドはオーバーフローを検出した場合、近似値(通常 infinity または -infinity)を返します。
- このメソッドはアンダーフローを検出した場合、0 を返します。
error-if-over-or-underflow? はキーワード引数です。
error-if-over-or-underflow? を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:
error-if-over-or-underflow? = false)。
StringInterface.to-int も参照してください。
戻り値
次の値を左から右の順に返します。
- 解析後の数値が格納された double。double の解析に失敗した場合は、0 を返します。
- このメソッドが解析した文字数を示す int 値。
- オーバーフローまたはアンダーフローが発生したかどうかを示す bool 値。この値が true の場合、オーバーフローまたはアンダーフローが発生しました。
説明
self は次の形式で指定します。
spaces[sign]num[exp]
説明:
文字列の解析例を次に示します。
- "365" は 365 になります。
- " 3.65" は 3.65 になります。
- "-365" は -365 になります。
- "365e10" は 3.65e+012 になります。
- " 365e-10" は 3.65e-008 になります。
文字列内の数値の後ろに文字を含めることができます。これらの文字は終了文字と呼ばれることもあります。このメソッドでは、終了文字は無視されます。ただし、解析された文字数を示す戻り値には、終了文字の字数も含まれます。たとえば、
"365e-10 is the multiplier" という文字列はこのメソッドのパラメータとして有効です。
self 内の文字を指定する際にこの書式を順守しなかった場合、倍精度型の数値として
0 が返されます。
例
次の例は、空白、数値、および負の指数から成る文字列を倍精度型の数値に変換するコードです。
例 |
 |
{value
|| Declare and initialize a string
let s:String = " 365e-10"
|| Declare variables to hold the return values from
|| a call to "to-double"
let number:double
let chars:int
let over-or-underflow?:bool
|| Call "to-double" and assign the multiple return
|| values to the variables.
set (number, chars, over-or-underflow?) =
{s.to-double error-if-over-or-underflow? = false}
|| Display a message containing the result of a call
|| to "to-double".
|| Note that you must use "value" to display the values
|| of the variables.
{text The number is {value number}, which occupied
{value chars} characters in the original string.}
|| Note that you can use "format" to change how Curl
|| displays numbers.
}
| |
次の例は、終了文字を含む文字列を倍精度型の数値に変換するコードです。
例 |
 |
{value
|| Declare and initialize a string
let s:String = "365e-10 is the multiplier"
|| Declare variables to hold the return values from
|| a call to "to-double"
let number:double
let chars:int
let over-or-underflow?:bool
|| Call "to-double" and assign the multiple return
|| values to the variables.
set (number, chars, over-or-underflow?) =
{s.to-double error-if-over-or-underflow? = false}
|| Display a message containing the result of a call
|| to "to-double".
{text The number is {value number}. {value chars}
characters were parsed.}
}
| |
次の例は、1 番目の戻り値だけを使うコードです。この方法は一般的なものです。
例 |
 |
{value
|| Declare and initialize a string
let s:String = "365e-10 is the multiplier"
|| Declare variable to hold the return value from
|| a call to "to-double"
let number:double
|| Call "to-double" and assign the first return
|| value to the variable.
set number = {s.to-double}
|| Display a message containing the result of a call
|| to "to-double".
{text The number is {value number}.}
}
| |
(メソッド)
self を格納する入力ストリームを返します。
戻り値
説明
例
次の例では、
to-InputStream メソッドを使用して文字列を入力ストリームに変換しています。
例 |
 |
{value
|| Declare and initialize a string.
let s:String = "Hello World!"
|| Use to-InputStream to set the contents of "s"
|| to the input stream.
let tis:TextInputStream = {s.to-InputStream}
|| Retrieve (and display) a string from the input
|| stream.
{tis.read-one-string}
}
| |
注意事項
(メソッド)
public | {StringInterface.to-int radix:int = 0,start:int = 0,whitespace:CharClass = CharClass.whitespace-chars,error-if-overflow?:bool = true }:(
val:int,
n-chars-consumed:int,
overflow?:bool,
radix:int
) |
self を解析して int 値にします。
radix: self 内における数値の記数法。基数 2 ~ 36 の範囲の記数法を指定できます。radix の既定値は 0 です。これは、self の中で記数法を指定することを意味します。つまり、10 進法以外の記数法を使う場合、self の中でその記数法のプレフィックスを指定します。有効な記数法プレフィックスは、2 進法の場合は 0b または 0B、8 進法の場合は 0o または 0O、16 進法の場合は 0x または 0X です。radix はキーワード引数です。radix を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:radix = 16)。
start:
self 内の解析開始位置。最左端の文字の位置は
0 です。最右端の文字の位置は
(self.size - 1) です。有効値は
0 ~
self.size の範囲です (
0 と
self.size を含む)。既定値は
0 です。
start はキーワード引数です。
start を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:
start = 4)。
start が範囲外を指している場合、このメソッドは
ArrayBoundsException をスローします。
whitespace:
self の先頭にある、空白を構成する文字。空白文字は通常、スペースとタブです。
whitespace の既定値は、現在のロケールに対する空白 (
CharClass.whitespace-chars で定義) を意味する文字群です。このメソッドの呼び出しに対して既定値以外の空白文字を指定するには、その空白文字を含む
CharClass を指定します。
whitespace はキーワード引数です。
whitespace を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:
whitespace = {CharClass " .,;:"})。空白文字以外にも無視したい文字がある場合、このパラメータを使ってその文字を指定できます。
error-if-overflow?:
数値が int 型の有効範囲外にある場合 (つまり、数値がオーバーフローした場合) にこのメソッドがエラーを生成するかどうかを示す、ブール値のフラグ。このメソッドは、10 進数に対しては符号付きオーバーフロー検査、その他の数値に対しては符号なしオーバーフロー検査を実行します。
error-if-overflow? を true に設定した場合、このメソッドはオーバーフロー検出時にエラーをスローします。エラーが発生するとプログラムの実行が停止し、エラー メッセージが表示されます。error-if-overflow? を false に設定した場合、このメソッドはエラーを生成せず、生成された値を返します。その際に、主要ビットが失われることになります。error-if-overflow? の既定値は true です。
error-if-overflow? はキーワード引数です。error-if-overflow? を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:error-if-overflow? = false)。
戻り値
次の値を左から右の順に返します。
- 解析後の整数を示す int 値。解析に失敗した場合は、0 を返します。
- このメソッドが解析した文字数を示す int 値。
- オーバーフローが発生したかどうかを示す bool 値。この値が true の場合、オーバーフローが発生しました。
- 数値の解析に使用された基数。
説明
self は次の形式で指定します。
spaces[sign][num-sys]num
説明:
- spaces:0 個以上の空白文字。whitespace パラメータで定義されたもの。
- sign:数値の符号 ("+" または"-"、オプション)。
- num-sys:記数法のプレフィックス (オプション)。有効な記数法プレフィックスは、2 進法の場合は 0b または 0B、8 進法の場合は 0o または 0O、16 進法の場合は 0x または 0X です。
- num :1 桁以上の数値。
文字列の解析例を次に示します。
- "365" は 365 (10 進表記) になります。
- "365" は 365 (10 進表記) になります。
- "-365" は -365 (10 進表記) になります。
- "0b101101101" は 365 (10 進表記) になります。
- " 0X16D" は 365 (10 進表記) になります。
文字列内の整数の後ろに文字を含めることができます。これらの文字は終了文字と呼ばれることもあります。このメソッドでは、終了文字は無視されます。ただし、解析された文字数を示す戻り値には、終了文字の字数も含まれます。たとえば、
"365 days of the year" という文字列はこのメソッドのパラメータとして有効です。
self 内の文字を指定する際にこの書式を順守しなかった場合、整数の値として
0 が返されます。
self の中で記数法プレフィックスを指定する場合、
radix パラメータもその記数法に合わせて設定してください。
StringInterface.to-int64 と
StringInterface.to-double も参照してください。
例
次の例は、空白、符号、および数値 (16 進表記) を整数に変換するコードです。
例 |
 |
{value
|| Declare and initialize a string
let s:String = " -0X16D"
|| Declare variables to hold the return values from
|| a call to "to-int"
let number:int
let chars:int
let overflow?:bool
|| Call "to-int" and assign the multiple return
|| values to the variables.
set (number, chars, overflow?) =
{s.to-int error-if-overflow? = false}
|| Display a message containing the result of a call
|| to "to-int".
|| Note that you must use "value" to display the values
|| of the variables.
{text The number is {value number}. {value chars}
characters were parsed.}
|| Note that to display an integer in a number system
|| other than decimal, you must use "format".
}
| |
次の例は、終了文字を含む文字列を整数型の数値に変換するものです。
例 |
 |
{value
|| Declare and initialize a string
let s:String = "365 days of the year"
|| Declare variables to hold the return values from
|| a call to "to-int"
let number:int
let chars:int
let overflow?:bool
|| Call "to-int" and assign the multiple return
|| values to the variables.
set (number, chars, overflow?) =
{s.to-int error-if-overflow? = false}
|| Display a message containing the result of a call
|| to "to-int".
{text The number is {value number}. {value chars}
characters were parsed.}
}
| |
次の例は、1 番目の戻り値だけを使うコードです。この方法は一般的なものです。
例 |
 |
{value
|| Declare and initialize a string
let s:String = "365 days of the year"
|| Declare a variable to hold the first return value
|| from a call to "to-int"
let number:int
|| Call "to-int" and assign the first return
|| value to the variable.
set number = {s.to-int}
|| Display a message containing the result of a call
|| to "to-int".
{text The number is {value number}.}
}
| |
(メソッド)
public | {StringInterface.to-int64 radix:int = 0,start:int = 0,whitespace:CharClass = CharClass.whitespace-chars,error-if-overflow?:bool = true }:(
val:int64,
n-chars-consumed:int,
overflow?:bool,
radix:int
) |
self を解析して int64 値にします。
説明
(メソッド)
public | {StringInterface.to-lower-clone}:String |
self のクローンを返します。文字は小文字に変換されます。
戻り値
例
例 |
 |
{value
|| Declare and initialize a string
let s1:String = "Hello World!"
|| Initialize s2 with a string containing the
|| characters of s1 in lowercase.
let s2:String = {s1.to-lower-clone}
|| Display the contents of s2.
{value s2}
}
| |
注意事項
クローン作成の詳細については、『Curl 開発者ガイド』の「
文字列クローンの操作」のセクションを参照してください。
(メソッド)
public | {StringInterface.to-String}:String |
self と同一 (==) の String を作成します。
戻り値
例
例 |
 |
{value
|| Define and initialize a StringBuf.
let sb:StringBuf = {StringBuf "Hello World!"}
|| Convert the StringBuf to a String.
let s:String = {sb.to-String}
|| Display the contents of the String.
{value s}
}
| |
(メソッド)
public | {StringInterface.to-upper-clone}:String |
self のクローンを返します。文字は大文字に変換されます。
戻り値
例
例 |
 |
{value
|| Declare and initialize a string
let s1:String = "Hello World!"
|| Initialize s2 with a string containing the
|| characters of s1 in uppercase.
let s2:String = {s1.to-upper-clone}
|| Display the contents of s2.
{value s2}
}
| |
注意事項
クローン作成の詳細については、『Curl 開発者ガイド』の「
文字列クローンの操作」のセクションを参照してください。
(メソッド)
public | {StringInterface.trim-clone trim-chars:CharClass = CharClass.whitespace-chars }:String |
self のクローンを返します。その際、文字列の左端と右端の両方がトリミングされます。
trim-chars: トリミングされる文字。
trim-chars の既定値は、現在のロケールに対する空白 (
CharClass.whitespace-chars で定義) を意味する文字群です。このメソッド呼び出しに対して既定値以外のトリミング対象文字を指定するには、その文字を含む
CharClass を指定します。
trim-chars はキーワード引数です。
trim-chars を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:
trim-chars = {CharClass " .,;:"})。
戻り値
説明
trim-chars で指定された文字を、self の左端から順に削除します。この処理を、trim-chars で指定されていない文字が出現するまで続けます。同じ処理を self の右端に対しても実行します。トリミング後の文字列を返します。
trim-chars で指定した文字がトリミング対象外の文字の間に出現した場合は、削除されません。たとえば、スペースがトリミング対象文字になっている場合、文字列の先頭と末尾にあるスペースは削除されますが、単語の間にあるスペースは削除されません。
例
例 |
 |
{value
|| Declare and initialize a string
let s1:String = " Hello World! "
|| Initialize s2 with the characters of s1,
|| removing whitespace from the beginning
|| and the end of the string.
let s2:String = {s1.trim-clone}
|| Display the contents of s2.
|| The # characters are used to indicate
|| beginning and end of the string.
{pre #{value s2}#}
}
| |
注意事項
クローン作成の詳細については、『Curl 開発者ガイド』の「
文字列クローンの操作」のセクションを参照してください。
(メソッド)
public | {StringInterface.trim-left-clone trim-chars:CharClass = CharClass.whitespace-chars }:String |
self のクローンを返します。その際、文字列の左端だけがトリミングされます。
trim-chars: トリミングされる文字。
trim-chars の既定値は、現在のロケールに対する空白 (
CharClass.whitespace-chars で定義) を意味する文字群です。このメソッド呼び出しに対して既定値以外のトリミング対象文字を指定するには、その文字を含む
CharClass を指定します。
trim-chars はキーワード引数です。
trim-chars を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:
trim-chars = {CharClass " .,;:"})。
戻り値
説明
trim-chars で指定された文字を、self の左端から順に削除します。この処理を、trim-chars で指定されていない文字が出現するまで続けます。トリミング後の文字列を返します。
例
例 |
 |
{value
|| Declare and initialize a string
let s1:String = " Hello World! "
|| Initialize s2 with the characters of s1,
|| removing whitespace from the beginning
|| of the string.
let s2:String = {s1.trim-left-clone}
|| Display the contents of s2.
|| The # characters are used to indicate
|| beginning and end of the string.
{pre #{value s2}#}
}
| |
注意事項
クローン作成の詳細については、『Curl 開発者ガイド』の「
文字列クローンの操作」のセクションを参照してください。
(メソッド)
public | {StringInterface.trim-right-clone trim-chars:CharClass = CharClass.whitespace-chars }:String |
self のクローンを返します。その際、文字列の右端だけがトリミングされます。
trim-chars: トリミングされる文字。
trim-chars の既定値は、現在のロケールに対する空白 (
CharClass.whitespace-chars で定義) を意味する文字群です。このメソッド呼び出しに対して既定値以外のトリミング対象文字を指定するには、その文字を含む
CharClass を指定します。
trim-chars はキーワード引数です。
trim-chars を指定するには、メソッド呼び出しのキーワードに対して適切な値を設定します (例:
trim-chars = {CharClass " .,;:"})。
戻り値
説明
trim-chars で指定された文字を、self の右端から順に削除します。この処理を、trim-chars で指定されていない文字が出現するまで続けます。トリミング後の文字列を返します。
例
例 |
 |
{value
|| Declare and initialize a string
let s1:String = " Hello World! "
|| Initialize s2 with the characters of s1,
|| removing whitespace from the end of the
|| string.
let s2:String = {s1.trim-right-clone}
|| Display the contents of s2.
|| The # characters are used to indicate
|| beginning and end of the string.
{pre #{value s2}#}
}
| |
注意事項
クローン作成の詳細については、『Curl 開発者ガイド』の「
文字列クローンの操作」のセクションを参照してください。